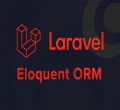
One to Many Polymorphic Model Relationship được sử dụng khi một model thuộc về nhiều model khác trên một model kết hợp duy nhất. Ví dụ: Nếu chúng ta có bảng post và video, cả hai đều cần thêm hệ thống nhận xét và bạn muốn có thể quản lý comment trong một bảng duy nhất cho cả post và video.
Vì vậy, trong hướng dẫn này, tôi sẽ hướng dẫn bạn tạo migrate, tạo dữ liệu và truy xuất dữ liệu trong One to Many Polymorphic Model Relationship.
Trong ví dụ này, tôi sẽ tạo bảng "posts", "videos" và "comments", mỗi bảng đều được liên kết với nhau. Bây giờ chúng ta sẽ tạo One to Many Polymorphic Model Relationship bằng cách sử dụng laravel Eloquent Model. Đầu tiên chúng ta sẽ tạo migrate, model, truy xuất dữ liệu và sau đó là cách tạo dữ liệu. Vì vậy, chúng ta sẽ thử một làm một ví dụ với cấu trúc bảng cơ sở dữ liệu như sau:
One to Many Polymorphic Relationship sẽ sử dụng "morphMany()" and "morphTo()" cho mối quan hệ.
Tạo migrations
Bây giờ chúng ta phải tạo migrate bảng posts, bảng videos và bảng comments. Bạn hãy tạo như hướng dẫn dưới đây:
Migration bảng posts
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string("name");
$table->timestamps();
});
Migration bảng videos
Schema::create('videos', function (Blueprint $table) {
$table->id();
$table->string("name");
$table->timestamps();
});
Migration bảng comments
Schema::create('comments', function (Blueprint $table) {
$table->id();
$table->string("body");
$table->integer('commentable_id');
$table->string("commentable_type");
$table->timestamps();
});
Tạo models
Tại đây, chúng ta sẽ tạo model bảng post, video và comment. chúng ta cũng sẽ sử dụng "morphMany ()" và "morphTo ()" cho mối quan hệ của cả hai model.
Model bảng posts
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
/**
* Get all of the post's comments.
*/
public function comments()
{
return $this->morphMany(Comment::class, 'commentable');
}
}
Model bảng videos
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Video extends Model
{
/**
* Get all of the post's comments.
*/
public function comments()
{
return $this->morphMany(Comment::class, 'commentable');
}
}
Model bảng comments
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Comment extends Model
{
/**
* Get all of the owning commentable models.
*/
public function commentable()
{
return $this->morphTo();
}
}
Truy vấn dữ liệu
$post = Post::find(1);
dd($post->comments);
$video = Video::find(1);
dd($video->comments);
Tạo mới dữ liệu
$post = Post::find(1);
$comment = new Comment;
$comment->body = "Hi ManhDanBlog";
$post->comments()->save($comment);
$video = Video::find(1);
$comment = new Comment;
$comment->body = "Hi ManhDanBlog";
$video->comments()->save($comment);
Tôi hy vọng bạn thích hướng dẫn này. Nếu bạn có bất kỳ câu hỏi nào hãy liên hệ với chúng tôi qua trang contact. Cảm ơn bạn.