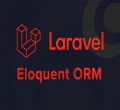
Nếu bạn có một form để người dùng nhập dữ liệu và bạn muốn kiểm tra dữ liệu đầu vào trước khi lưu xuống database chẳng hạn thì bạn có 2 cách sau đây:
Cách 1: Bạn thêm validate trực tiếp vào hàm store hoặc update bạn có thể xem lại bài viết này.
Cách 2: Bạn tạo một class request riêng để kiểm tra dữ liệu.
Cá nhân mình thì mình sẽ chọn cách 2 vì điều này giúp cho controller trở nên sạch hơn một chút và các quy tắc kiểm tra dữ liệu sẽ nằm ở một nơi.
Bây giờ, chúng ta sẽ bắt đầu tìm hiểu cách hoạt động của nó như thế nào thông qua ví dụ sau đây.
Giả sử, chúng ta sẽ tạo một form gồm có những thông tin title và body.
Đầu tiên, chúng ta cần tạo một controller mới bằng lệnh command sau đây:
php artisan make:controller PostController
Tiếp theo, chúng ta cần phải tạo mới một route có url là "/post" có 2 method là get và post, bạn mở file routes/web.php và chỉnh sửa như sau:
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\PostController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('post', [PostController::class, 'create'])->name('posts.create');
Route::post('post', [PostController::class, 'store'])->name('posts.store');
Tiếp theo, chúng ta sẽ tạo ra class request có tên là StorePostRequest bằng lệnh command sau đây:
php artisan make:request StorePostRequest
Sau khi lệnh command trên chạy xong, nó tạo ra file StorePostRequest.php nằm trong thư mục app/Http/Requests, bạn hãy mở file StorePostRequest.php và chỉnh sửa như sau:
<?php
namespace App\Http\Requests;
use Illuminate\Foundation\Http\FormRequest;
class StorePostRequest extends FormRequest
{
/**
* Determine if the user is authorized to make this request.
*
* @return bool
*/
public function authorize()
{
return true;
}
/**
* Get the validation rules that apply to the request.
*
* @return array
*/
public function rules()
{
return [
'title' => 'required|max:255',
'body' => 'required',
];
}
}
Tiếp theo, chúng ta sẽ sử dụng class request vừa mới tạo ở controller như sau:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Requests\StorePostRequest;
class PostController extends Controller
{
public function create()
{
return view('post');
}
function store(StorePostRequest $request)
{
// store your post data
}
}
Bây giờ, chúng ta hãy tạo file post.blade.php trong thư mục resources/views
<!DOCTYPE html>
<html lang="en">
<head>
<title>Bootstrap Example</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container">
<h2>Laravel Validation ManhDanBlogs</h2>
<form method="post">
@csrf
@if ($errors->any())
<div class="alert alert-danger">
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
<div class="form-group">
<label for="title">Title:</label>
<input type="text" class="form-control" name="title" value="{{ old("title") }}">
</div>
<div class="form-group">
<label for="pwd">Body:</label>
<textarea class="form-control" name="body">{{ old("body") }}</textarea>
</div>
<button type="submit" class="btn btn-default">Submit</button>
</form>
</div>
</body>
</html>
Đoạn mã dưới đây dùng để hiển thị các thông báo lỗi
@if ($errors->any())
<div class="alert alert-danger">
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
Bây giờ, bạn hãy nhìn tổng quan thì mã nguồn của chúng ta đẹp hơn rồi phải không?
Customization Class Request
Custom Messages
Bây giờ, điều gì sẽ xảy ra nếu bạn muốn thay đổi các thông báo của class request?
Thật dễ dàng để thực hiện với hàm messages() trong class request. Bạn chỉ cần xác định một mảng, trong đó key là tên field và value là thông bào bạn muốn thay đổi
<?php
namespace App\Http\Requests;
use Illuminate\Foundation\Http\FormRequest;
class StorePostRequest extends FormRequest
{
/**
* Determine if the user is authorized to make this request.
*
* @return bool
*/
public function authorize()
{
return true;
}
/**
* Get the validation rules that apply to the request.
*
* @return array
*/
public function rules()
{
return [
'title' => 'required|max:255',
'body' => 'required',
];
}
/**
* Custom message for validation
*
* @return array
*/
public function messages()
{
return [
"title.required" => "Please write a title",
"title.max" => "The title has to have no more than :max characters.",
"body.required" => "Please write some content",
];
}
}
Special Naming for Attributes
Như bạn đã biết, Laravel sẽ tự động tạo ra các câu thông báo validation. Vì vậy, đôi khi chúng ta cần cung cấp các tên đặc biệt cho các thuộc tính của mình. Bạn có thể sử dụng hàm attributes để thay đổi tên các thuộc tính để hiển thị những câu thông báo thân thiện hơn với người dùng, trong đó key là tên field, value là tên field mà bạn muốn thay thế.
<?php
namespace App\Http\Requests;
use Illuminate\Foundation\Http\FormRequest;
class StorePostRequest extends FormRequest
{
/**
* Determine if the user is authorized to make this request.
*
* @return bool
*/
public function authorize()
{
return true;
}
/**
* Get the validation rules that apply to the request.
*
* @return array
*/
public function rules()
{
return [
'title' => 'required|max:255',
'body' => 'required',
];
}
/**
* Get custom attributes for validator errors.
*
* @return array
*/
public function attributes()
{
return [
"body" => "Another Name",
];
}
}
Tôi hy vọng bạn thích hướng dẫn này. Nếu bạn có bất kỳ câu hỏi nào hãy liên hệ với chúng tôi qua trang contact. Cảm ơn bạn.