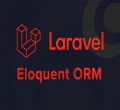
Mối quan hệ một-một là một mối quan hệ rất cơ bản. Trong hướng dẫn này, tôi sẽ hướng dẫn bạn cách tạo dữ liệu và truy xuất dữ liệu bằng Eloquent Model.
Trong hướng dẫn này, tôi sẽ tạo hai bảng là users và phones với cấu trúc cơ sở dữ liệu như sau:
One to One Relationship sẽ sử dụng "hasOne()" và "belongsTo()" cho mối quan hệ.
Tạo Migrations
Bây giờ chúng ta phải tạo migrate cho bảng users, bảng phones và thêm foreign key với bảng users. Vì vậy, bạn hãy tạo như hướng dẫn dưới đây:
Migration bảng users
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->timestamp('email_verified_at')->nullable();
$table->string('password');
$table->rememberToken();
$table->timestamps();
});
Migration bảng phones
Schema::create('phones', function (Blueprint $table) {
$table->id();
$table->unsignedBigInteger('user_id');
$table->string('phone');
$table->timestamps();
$table->foreign('user_id')->references('id')->on('users')->onDelete('cascade');
});
Tạo Models
Ở đây, chúng ta sẽ tạo model bảng users và phones. Chúng ta cũng sẽ sử dụng "hasOne ()" và "belongsTo()" cho mối quan hệ của cả hai model.
Model bảng users
<?php
namespace App\Models;
use Illuminate\Notifications\Notifiable;
use Illuminate\Foundation\Auth\User as Authenticatable;
class User extends Authenticatable
{
use Notifiable;
/**
* The attributes that are mass assignable.
*
* @var array
*/
protected $fillable = [
'name', 'email', 'password',
];
/**
* The attributes that should be hidden for arrays.
*
* @var array
*/
protected $hidden = [
'password', 'remember_token',
];
/**
* Get the phone record associated with the user.
*/
public function phone()
{
return $this->hasOne(Phone::class);
}
}
Model bảng phones
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Phone extends Model
{
/**
* Get the user that owns the phone.
*/
public function user()
{
return $this->belongsTo(User::class);
}
}
Truy vấn dữ liệu
$phone = User::find(1)->phone;
dd($phone);
$user = Phone::find(1)->user;
dd($user);
Tạo mới dữ liệu
$user = User::find(1);
$phone = new Phone;
$phone->phone = '9429343852';
$user->phone()->save($phone);
$phone = Phone::find(1);
$user = User::find(10);
$phone->user()->associate($user)->save();
Tôi hy vọng bạn thích hướng dẫn này. Nếu bạn có bất kỳ câu hỏi nào hãy liên hệ với chúng tôi qua trang contact. Cảm ơn bạn.