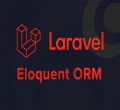
Trong mô hình MVC, chữ "C" là từ viết tắt của Controller và nó đóng vai trò rất quan trọng để phân tích các logic business. Khi người dùng truy cập vào trình duyệt, nó sẽ đi đến route đầu tiên, sau đó nó sẽ tìm thấy controller của route đó.
Controller sẽ nhận một số dữ liệu cần thiết để phản hồi thông qua View. Khi làm việc với Laravel Controller, điều rất quan trọng là phải biết tất các phương thức cũng như chức năng có sẵn mà bạn có thể sử dụng khi đang ở Controller.
Tạo controller
Để tạo controller, bạn hãy mở command line ở thư mục root Laravel. Khi đó, bạn có thể chạy kì lệnh nào sau đây để tạo controller
# Create a simple blank controller
php artisan make:controller UserController
# Create resource full controller with CRUD methods
php artisan make:controller UserController --resource
# Create resource full controller with CRUD API methods
php artisan make:controller UserController --api
Sau khi chạy xong lệnh tạo, một controller mới được tạo ở app/Http/Controllers/UserController.php.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UserController extends Controller
{
}
Controller có thể chứa các phương thức khác nhau, mỗi phương thức có thể có tham số $request. Về cơ bản, có thể nó chứa variables, session hoặc cookie.
Mỗi function trong controller có thể phản hồi khác nhau, sau đây là một số ví dụ
Render plain text
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UserController extends Controller
{
function example()
{
return "Hello, Sandip Patel";
}
}
Render view
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UserController extends Controller
{
function example()
{
return view("dashboard");
}
}
Render array as json or json array
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UserController extends Controller
{
function example()
{
// render array as json
return [1, 2, 3];
// render json array
return response()->json([
"name" => "ManhDanBlogs"
]);
}
}
Render text kèm theo headers
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UserController extends Controller
{
function example()
{
return response('ManhDanBlogs', 200)
->header('Content-Type', 'text/plain');
return response($content)
->header('Content-Type', $type)
->header('X-Header-One', 'Header Value1')
->header('X-Header-Two', 'Header Value2');
}
}
Render file
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UserController extends Controller
{
function example()
{
return response()->file($pathToFile);
}
}
Render file download
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UserController extends Controller
{
function example()
{
return response()->download($pathToFile);
}
}
Redirect
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UserController extends Controller
{
function example()
{
// redirect to last page with
// submitted form data
return back()->withInput();
// redirect to user/dashboard page
return redirect('user/dashboard');
// redirect with named route
return redirect()->route('login');
// redirect with named route by passing variables
return redirect()->route('profile', ['id' => 1]);
// redirect to specific controller method
return redirect()->action('HomeController@index');
// redirect to external url
return redirect()->away('https://www.google.com');
// redirect with flash session message
return redirect('dashboard')->with('success', 'Profile updated!');
}
}
Sessions
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UserController extends Controller
{
function example(Request $request)
{
// get all session data
$data = $request->session()->all();
// get a name variable from session store
$name = $request->session()->get('name');
// get a name from the session store if not available return default name
$name = $request->session()->get('name', 'ManhDanBlogs');
// store provided name in session store
$request->session()->put('name', "ManhDanBlogs");
// push a new item to array in session store
$request->session()->push('user.teams', 'developers');
// flush the session data
$request->session()->flush();
// re-generate new session id
$request->session()->regenerate();
// delete data from the session
$request->session()->forget('key');
$request->session()->forget(['key1', 'key2']);
// create a new flash session variable to use in view file
$request->session()->flash('success', 'Task was successful!');
// get the data from the session and delete the key afterwards
$value = $request->session()->pull('key', 'default');
// check to see if item present in the session
// it returns true if item does exist with not null value
if ($request->session()->has('users')) {
//
}
// check to see if item present in the session
// returns true if item does exists with or without null value
if ($request->session()->exists('users')) {
//
}
// get a piece of data from the session
// using global helper function
$name = session('name');
// Specifying a default value...
// using global helper function
$name = session('name', 'default');
// store session data
session(['key' => 'value']);
}
}
Cookies
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Cookie;
class UserController extends Controller
{
function example(Request $request)
{
// get cookie from request
$value = $request->cookie('name');
// get cookie using facade
$value = Cookie::get('name');
// create a new cookie that lasts for next 5 minutes
// response data with newly created cookie
return response()->withCookie(cookie('name', 'ManhDanBlogs', 5));
}
}
Form data
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UserController extends Controller
{
function example(Request $request)
{
// get all form data
$input = $request->all();
// get the name from the form data
$name = $request->input('name');
// get name field from the form and pass default value
$name = $request->input('name', 'ManhDanBlogs');
// access array key from form data
$name = $request->input('products.0.name');
$names = $request->input('products.*.name');
// access json key from the request data
$name = $request->input('user.name');
// get all fields except credit_card field
$input = $request->except('credit_card');
// fetch only few keys from the form data
$input = $request->only(['username', 'password']);
// get all query variables
$query = $request->query();
// get name from querystring
$name = $request->query('name');
$name = $request->query('name', 'ManhDanBlogs');
// The old method will pull the previously flashed input data from the session:
$username = $request->old('username');
// has method returns true if the value is present on the request:
if ($request->has('name')) {
//
}
// check if multiple fields are present
if ($request->has(['name', 'email'])) {
//
}
// The hasAny method returns true if any of the specified values are present:
if ($request->hasAny(['name', 'email'])) {
//
}
// If you would like to determine if a value is present on the request, not empty
if ($request->filled('name')) {
//
}
// To determine if a given key is absent from the request
if ($request->missing('name')) {
//
}
// get file field
$file = $request->file('photo');
// check if file uploaded is valid file
if ($request->file('photo')->isValid()) {
//
}
// get path and extension of a file
$path = $file->path();
$extension = $file->extension();
// store file in storage/images folder
$path = $file->store('images');
// store file with different name in storage/images
$path = $file->storeAs('images', 'filename.jpg');
}
}
Tôi hy vọng bạn thích hướng dẫn này. Nếu bạn có bất kỳ câu hỏi nào hãy liên hệ với chúng tôi qua trang contact. Cảm ơn bạn.