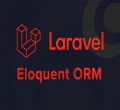
Đôi khi trong dự án, chúng ta cần xác định thời lượng video được phép upload lên server. Nhưng rất tiếc, Laravel không cung cấp validate xác định thời lượng video để chúng ta thực hiện được. Vì vậy, trong bài viết này tôi sẽ hướng dẫn các bạn tạo ra một custom rule để thực hiện việc xác định thời lượng và min - max thời lượng video được phép upload.
Trước khi bắt đầu, bạn cần đảm bảo môi trường linux của bạn đã cài package FFmpeg.
Đầu tiên, chúng ta sẽ cài package pbmedia/laravel-ffmpeg để hỗ trợ chúng ta lấy được những thông tin video cần thiết, bạn hãy chạy lệnh command sau đây:
composer require pbmedia/laravel-ffmpeg
Tiếp theo, chúng ta sẽ thêm Service Provider and Facade vào file config/app.php
'providers' => [
...
ProtoneMedia\LaravelFFMpeg\Support\ServiceProvider::class,
...
];
'aliases' => [
...
'FFMpeg' => ProtoneMedia\LaravelFFMpeg\Support\FFMpeg::class
...
];
Tiếp theo, chúng ta sẽ tạo file config bằng lệnh command sau đây:
php artisan vendor:publish --provider="ProtoneMedia\LaravelFFMpeg\Support\ServiceProvider"
Bạn hãy thêm FFMPEG_BINARIES và FFPROBE_BINARIES vào file .env như sau:
FFMPEG_BINARIES=/usr/bin/ffmpeg
FFPROBE_BINARIES=/usr/bin/ffprobe
Tiếp theo, bạn hãy thêm config disk vào file config/filesystems.php như sau:
'disks' => [
....
'ffmpeg' => [
'driver' => 'local',
'root' => '/',
],
],
Vậy là quá trình chuẩn bị đã hoàn tất, tiếp theo chúng ta sẽ tạo một custom rule tên là VideoLength bằng lệnh command sau đây:
php artisan make:rule VideoLength
Sau khi lệnh command trên chạy xong, một file mới tên là VideoLength.php nằm ở thư mục app/Rules, bạn hãy mở file và chỉnh sửa như sau:
<?php
namespace App\Rules;
use Illuminate\Contracts\Validation\Rule;
use FFMpeg;
class VideoLength implements Rule
{
public $min;
public $max;
public $duration;
/**
* Create a new rule instance.
*
* @return void
*/
public function __construct($min = 0, $max = PHP_INT_MAX)
{
$this->min = $min;
$this->max = $max;
}
/**
* Determine if the validation rule passes.
*
* @param string $attribute
* @param mixed $value
* @return bool
*/
public function passes($attribute, $value)
{
$mime = $value->getMimeType() ?? "";
if(strstr($mime, "video/")) {
$duration = FFMpeg::fromDisk('ffmpeg')->open($value->getRealPath())->getDurationInSeconds();
$minFlag = $duration >= $this->min ? true : false;
$maxFlag = $duration <= $this->max ? true : false;
return $minFlag and $maxFlag;
}
return false;
}
/**
* Get the validation error message.
*
* @return string
*/
public function message()
{
if ($this->duration < $this->min) {
return "Video is smaller than {$this->min} minute. upload should be between {$this->min} - {$this->max} minute";
} else {
return "video is larger than {$this->max} minute. upload should be between {$this->min} - {$this->max} minute";
}
}
}
Như vậy, chúng ta đã tạo ra một rule để có thể giới hạn thời lượng của video, tôi hy vọng hướng dẫn của tôi sẽ giúp ích cho công việc của bạn. Nếu bạn có bất kỳ câu hỏi nào hãy liên hệ với chúng tôi qua trang contact. Cảm ơn bạn.