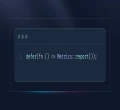
One to Many Relationship được sử dụng trong trường hợp một dữ liệu của một bảng được liên kết với một hoặc nhiều dữ liệu ở bảng khác. Ví dụ, một bài post có thể có nhiều comment.
Vì vậy, trong hướng dẫn này, tôi sẽ hướng dẫn bạn tạo migrate, tạo dữ liệu và truy xuất dữ liệu trong One to Many Relationship.
Trong ví dụ này, tôi sẽ tạo bảng "posts" và bảng "comments". cả hai bảng đều được liên kết với nhau. Bây giờ chúng ta sẽ tạo One to Many Relationship bằng cách sử dụng laravel Eloquent Model. Đầu tiên chúng ta sẽ tạo migrate, model, truy xuất dữ liệu và sau đó là cách tạo dữ liệu. Vì vậy, chúng ta sẽ thử một làm một ví dụ với cấu trúc bảng cơ sở dữ liệu như sau
One to Many Relationship sẽ sử dụng "hasMany()" and "belongsTo()" cho mối quan hệ.
Tạo Migrations
Bây giờ chúng ta phải tạo migrate cho bảng posts, bảng comments và thêm foreign key với bảng posts. Vì vậy, bạn hãy tạo như hướng dẫn dưới đây:
Migration bảng posts
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string("name");
$table->timestamps();
});
Migration bảng comments
Schema::create('comments', function (Blueprint $table) {
$table->id();
$table->unsignedBigInteger('post_id');
$table->string("comment");
$table->timestamps();
$table->foreign('post_id')->references('id')->on('posts')->onDelete('cascade');
});
Create Models
Tại đây, chúng ta sẽ tạo model bảng post và comment. Chúng ta cũng sẽ sử dụng "hasMany ()" và "belongsTo()" cho mối quan hệ của cả hai model.
Model bảng posts
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
/**
* Get the comments for the blog post.
*/
public function comments()
{
return $this->hasMany(Comment::class);
}
}
Model bảng comments
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Comment extends Model
{
/**
* Get the post that owns the comment.
*/
public function post()
{
return $this->belongsTo(Post::class);
}
}
Truy vấn dữ liệu
$post = Post::find(1);
$comments = $post->comments;
dd($comments);
$comment = Comment::find(1);
$post = $comment->post;
dd($post);
Tạo mới dữ liệu
$post = Post::find(1);
$comment = new Comment;
$comment->comment = "Hi ManhDanBlog";
$post = $post->comments()->save($comment);
$post = Post::find(1);
$comment1 = new Comment;
$comment1->comment = "Hi ManhDanBlog Comment 1";
$comment2 = new Comment;
$comment2->comment = "Hi ManhDanBlog Comment 2";
$post = $post->comments()->saveMany([$comment1, $comment2]);
$comment = Comment::find(1);
$post = Post::find(2);
$comment->post()->associate($post)->save();
Tôi hy vọng bạn thích hướng dẫn này. Nếu bạn có bất kỳ câu hỏi nào hãy liên hệ với chúng tôi qua trang contact. Cảm ơn bạn.