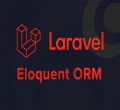
Trong thư viện laravel/ui, thì chức năng password reset dù cho token có hết hạn thì vẫn có truy cập vào trang password reset, đến khi bạn submit form thì mới thông báo là token đã hết hạn. Nhưng có một số dự án, thì người người ta muốn chuyển đến trang password expire chẳng hạn chứ không muốn người dùng submit form thì mới thông báo lỗi.
Trong bài viết này, tôi sẽ hướng dẫn các bạn chuyển hướng đến trang password expire khi token hết hạn.
Đầu tiên, chúng ta mở thư mục resources\views\auth\passwords và tạo một file có tên là expired.blade.php và có nội dung như sau:
@extends('layouts.app')
@section('content')
<div class="container">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="card">
<div class="card-header">{{ __('Password Reset') }}</div>
<div class="card-body">
{{ __('Link reset password has expired. Please try again.') }}
</div>
</div>
</div>
</div>
</div>
@endsection
Bạn mở file ResetPasswordController.php nằm trong thư mục app\Http\Controllers\Auth và chỉnh sửa như sau:
<?php
namespace App\Http\Controllers\Auth;
use App\Http\Controllers\Controller;
use App\Providers\RouteServiceProvider;
use Illuminate\Foundation\Auth\ResetsPasswords;
class ResetPasswordController extends Controller
{
/*
|--------------------------------------------------------------------------
| Password Reset Controller
|--------------------------------------------------------------------------
|
| This controller is responsible for handling password reset requests
| and uses a simple trait to include this behavior. You're free to
| explore this trait and override any methods you wish to tweak.
|
*/
use ResetsPasswords;
/**
* Where to redirect users after resetting their password.
*
* @var string
*/
protected $redirectTo = RouteServiceProvider::HOME;
/**
* Display the password expired view.
* @return \Illuminate\Contracts\View\Factory|\Illuminate\View\View
*/
public function expired()
{
return view('auth.passwords.expired');
}
}
Tiếp theo, bạn mở file web.php nằm trong thư mục routes và thêm dòng bên dưới:
Route::get('/passwords/expired', [App\Http\Controllers\Auth\ResetPasswordController::class, 'expired'])->name('passwords.expired');
Tiếp theo, chúng ta thực hiện chuyển hướng đến URL /passwords/expired nếu như token đã hết hạn sử dụng, bạn hãy mở file ResetPasswordController.php nằm trong thư mục app\Http\Controllers\Auth và chỉnh sửa như sau:
<?php
namespace App\Http\Controllers\Auth;
use App\Http\Controllers\Controller;
use App\Providers\RouteServiceProvider;
use Illuminate\Foundation\Auth\ResetsPasswords;
use Illuminate\Contracts\Hashing\Hasher as HasherContract;
use Illuminate\Http\Request;
use Carbon\Carbon;
use DB;
class ResetPasswordController extends Controller
{
/*
|--------------------------------------------------------------------------
| Password Reset Controller
|--------------------------------------------------------------------------
|
| This controller is responsible for handling password reset requests
| and uses a simple trait to include this behavior. You're free to
| explore this trait and override any methods you wish to tweak.
|
*/
use ResetsPasswords;
public function __construct(HasherContract $hasher)
{
$this->hasher = $hasher;
}
/**
* Where to redirect users after resetting their password.
*
* @var string
*/
protected $redirectTo = RouteServiceProvider::HOME;
/**
* Display the password reset view for the given token.
*
* If no token is present, display the link request form.
*
* @param \Illuminate\Http\Request $request
* @param string|null $token
* @return \Illuminate\Contracts\View\Factory|\Illuminate\View\View
*/
public function showResetForm(Request $request, $token = null)
{
$password_reset = DB::table("password_resets")->where('email', $request->email)->first();
if($password_reset && $token && $request->email && !$this->tokenExpired($password_reset->created_at) && $this->hasher->check($token, $password_reset->token) ) {
return view('auth.passwords.reset')->with(['token' => $token, 'email' => $request->email]);
}
return redirect()->route('passwords.expired');
}
/**
* Determine if the token has expired.
*
* @param string $createdAt
* @return bool
*/
protected function tokenExpired($createdAt)
{
return Carbon::parse($createdAt)->addMinutes(config('auth.passwords.users.expire'))->isPast();
}
/**
* Display the password expired view.
* @return \Illuminate\Contracts\View\Factory|\Illuminate\View\View
*/
public function expired()
{
return view('auth.passwords.expired');
}
}
Khi bạn truy cập trang URL password reset mà token hết hạn, nó sẽ chuyển hướng đến trang password expired và thông báo người dùng link đã không còn sử dụng được. Tôi hy vọng bạn thích hướng dẫn này. Nếu bạn có bất kỳ câu hỏi nào hãy liên hệ với chúng tôi qua trang contact. Cảm ơn bạn.