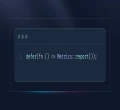
Trong quá trình phát triển web, việc gửi email là một chức năng quan trọng để thông báo, đặt lại mật khẩu, hoặc tương tác với người dùng.
Tuy nhiên, khi chúng ta đang trong quá trình phát triển, việc thử nghiệm và gỡ lỗi chức năng gửi email lại càng trở nên quan trọng hơn. Trong những trường hợp như vậy, chúng ta thường muốn tránh gửi email thực sự đến người dùng thực tế.
Laravel cung cấp một sự kiện có tên là MessageSending
cho phép chúng ta điều chỉnh hành vi gửi email.
Dựa trên sự kiện này, chúng ta sẽ tạo một Listener
có tên là MessageSendingRedirector
để điều hướng tất cả các email được gửi, đảm bảo rằng trong quá trình phát triển hoặc gỡ lỗi, tất cả các thông điệp email sẽ được chuyển đến địa chỉ email của đội ngũ nhà phát triển.
Tính năng này rất hữu ích khi làm việc với database của môi trường thực, nơi nhà phát triển muốn ngăn chặn email thử nghiệm đến người dùng thực tế.
Cấu hình Mail Sending Redirector
Bạn thêm các config bên dưới vào config/mail.php
:
/*
|--------------------------------------------------------------------------
| Email Redirection Settings
|--------------------------------------------------------------------------
|
| Configure the redirection settings for outgoing emails. These settings
| control whether the email redirection feature is enabled, and specify
| the recipients, reply-to address, and error-to address for redirection.
|
| 'enabled': Indicates whether the email redirection feature is active.
|
| 'to': Default recipient for redirected emails.
|
| 'cc': Default carbon copy (CC) recipient for redirected emails.
|
| 'bcc': Default blind carbon copy (BCC) recipient for redirected emails.
|
| 'reply_to': Default reply-to address for redirected emails.
|
| 'error_to': Default error-to address for redirection error notifications.
|
|--------------------------------------------------------------------------
*/
'redirect' => [
'enabled' => env('REDIRECT_MAIL_ENABLED', true),
'to' => env('REDIRECT_MAIL_TO', '[email protected]'),
'cc' => env('REDIRECT_MAIL_CC', '[email protected]'),
'bcc' => env('REDIRECT_MAIL_BCC', '[email protected]'),
'reply_to' => env('REDIRECT_MAIL_REPLY_TO', '[email protected]'),
'error_to' => env('REDIRECT_MAIL_ERROR_TO', '[email protected]'),
],
Listener MessageSendingRedirector
Để tạo listener MessageSendingRedirector
, bạn cần sử dụng lệnh sau:
php artisan make:listener MessageSendingRedirector
Sau đó, bạn hãy chỉnh sửa app/Listeners/MessageSendingRedirector.php
với nội dung như sau:
<?php
namespace App\Listeners;
use Illuminate\Mail\Events\MessageSending;
class MessageSendingRedirector
{
/**
* Handle the event.
*
* @param MessageSending $event
* @return void
*/
public function handle(MessageSending $event)
{
// Check if the email sending redirection feature is enabled
if (!$this->getConfigValue('enabled')) {
return; // If not, exit the function
}
// Set recipients for email sending redirection
$this->setRecipients($event);
// Set the Reply-To address for email sending redirection
$this->setReplyTo($event);
// Set the Errors-To header for email sending redirection
$this->setErrorTo($event);
}
/**
* Set the recipients for redirection.
*
* @param MessageSending $event
* @return void
*/
protected function setRecipients(MessageSending $event)
{
if ($event->message->getTo()) {
$event->message->setTo($this->getConfigValue('to'));
}
if ($event->message->getCc()) {
$event->message->setCc($this->getConfigValue('cc'));
}
if ($event->message->getBcc()) {
$event->message->setBCc($this->getConfigValue('bcc'));
}
}
/**
* Set the Reply-To address for redirection.
*
* @param MessageSending $event
* @return void
*/
protected function setReplyTo(MessageSending $event)
{
if ($event->message->getReplyTo()) {
$event->message->setReplyTo($this->getConfigValue('reply_to'));
}
}
/**
* Set the Errors-To header for redirection.
*
* @param MessageSending $event
* @return void
*/
protected function setErrorTo(MessageSending $event)
{
if ($event->message->getHeaders()->get('Errors-To')) {
$event->message->getHeaders()->get('Errors-To')->setValue($this->getConfigValue('error_to'));
}
}
/**
* Get configuration value and split it using '|' delimiter.
*
* @param string $key
* @return array
*/
protected function getConfigValue($key)
{
return explode('|', config("mail.redirect.$key"));
}
}
Chúng ta sẽ đăng ký MessageSendingRedirector
để lắng nghe sự kiện MessageSending
. Sự kiện này sẽ được kích hoạt khi một email được gửi.
Bạn hãy chỉnh sửa /app/Providers/EventServiceProvider.php
với nội dung như sau:
<?php
namespace App\Providers;
...
use Illuminate\Mail\Events\MessageSending;
use App\Listeners\MessageSendingRedirector;
class EventServiceProvider extends ServiceProvider
{
/**
* The event listener mappings for the application.
*
* @var array
*/
protected $listen = [
...
MessageSending::class => [
MessageSendingRedirector::class,
],
];
...
}
Cách sử dụng Mail Sending Redirector
Để sử dụng Mail Sending Redirector, bạn cần cấu hình các giá trị sau trong .env
của bạn:
REDIRECT_MAIL_ENABLED=true
REDIRECT_MAIL_TO="[email protected]"
REDIRECT_MAIL_CC="[email protected]"
REDIRECT_MAIL_BCC="[email protected]"
REDIRECT_MAIL_REPLY_TO="[email protected]"
REDIRECT_MAIL_ERROR_TO="[email protected]"
Nếu bạn muốn cấu hình chuyển hướng đến nhiều địa chỉ email khác nhau, bạn có thể sử dụng ký tự "|"
để phân tách chúng như sau:
REDIRECT_MAIL_ENABLED=true
REDIRECT_MAIL_TO="[email protected]|[email protected]"
REDIRECT_MAIL_CC="[email protected]|[email protected]"
REDIRECT_MAIL_BCC="[email protected]|[email protected]"
REDIRECT_MAIL_REPLY_TO="[email protected]|[email protected]"
REDIRECT_MAIL_ERROR_TO="[email protected]|[email protected]"